In the world of Java programming, Java strings are like the building blocks of communication. They represent sequences of characters or, simply put, character arrays. What makes strings unique in Java is that they are treated as objects. To navigate this realm effectively, Java provides the String class, a versatile tool for creating and manipulating strings. Let’s embark on a journey to explore Java strings, from their creation to understanding their immutability and the methods that empower you to work with them.
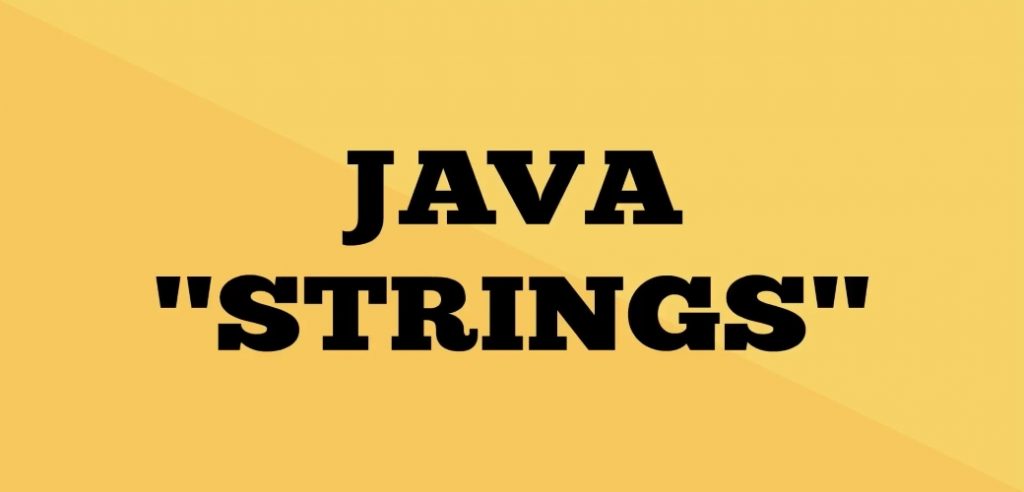
Creating a String: The Basics Java Strings
The most straightforward way to create a string in Java is by using string literals. For example:
String greeting = "Hello world!";
When the Java compiler encounters a string literal in your code, it automatically creates a String object with the specified value, such as “Hello world!”. But creating strings is not limited to literals; you can also use the new keyword and constructors provided by the String class. Java’s String class boasts eleven constructors, each allowing you to initialize a string using various sources, like character arrays.
In other words “Whenever it encounters a string literal in your code, the compiler creates a String object with its value in this case, “Hello world!’. As with any other object, you can create String objects by using the new keyword and a constructor. The String class has eleven constructors that allow you to provide the initial value of the string using different sources, such as an array of characters.”
Here’s an example of creating a string from a character array:
public class StringDemo{
public static void main(String args[]){
char[] helloArray = { 'h', 'e', 'l', 'l', 'o', '.'};
String helloString = new String(helloArray);
System.out.println(helloString);
}
}
This code snippet results in the output “hello.” It showcases how you can construct strings using character arrays.
Immutability: The Immutable Nature of Java Strings
One crucial aspect of Java strings is their immutability. Once a string is formed/created, its content remains unalterable or unchangable. This might sound counterintuitive, especially when you encounter string methods that appear to modify strings. However, what these methods do is create and return a new string that contains the result of the operation, leaving the original string untouched.
Accessor Methods: Understanding Java Strings
Strings provide a variety of methods to obtain information about them. These methods, known as accessor methods, empower you to understand and manipulate strings effectively. One such method is length(), which returns the number of characters contained in a string object.
For instance, after executing the following lines of code:
String palindrome = "Dot saw I was Tod";
int len = palindrome.length();
The variable len will hold the value 17, as there are 17 characters in the string “Dot saw I was Tod.”
Note on java Strings
The Java String class offers a range of methods for inspecting individual characters within a sequence, comparing strings, searching for substrings, extracting substrings, and transforming a string into uppercase or lowercase. The character casing operations adhere to the Unicode Standard, as specified by the Character class.
In the Java language, there is dedicated support for the string concatenation operator (+) and converting other objects into strings. For more details on string concatenation and conversion, you can refer to The Java™ Language Specification.
It’s essential to note that, unless explicitly mentioned, passing a null argument to a constructor or method within the String class will result in a NullPointerException being thrown.
In the UTF-16 format used by Java, a String represents a string, and supplementary characters are represented by surrogate pairs. This means that index values refer to char code units, so a supplementary character occupies two positions within a String.
The String class provides methods for working with Unicode code points (i.e., characters) in addition to those designed for Unicode code units (i.e., char values).
In general, methods for comparing Strings do not consider locale-specific factors. For more precise, locale-sensitive String comparisons, the Collator class offers relevant methods.
Conclusion – Java Strings
Java strings are more than just sequences of characters; they are essential objects for communication and data manipulation. Understanding how to create strings, recognizing their immutability, and leveraging accessor methods like length() are fundamental steps in mastering Java strings. With this knowledge, you can wield the power of strings effectively in your Java programs and explore the vast possibilities they offer.
Read other articles on Java Programming Language is here.
Official Documentation on Java.