What is Type Casting:
Type casting is the process of converting the value of one data type to another data type. It is used in computer programming to ensure that variables are correctly processed by a function or an expression. For example, type casting can be used to convert an integer to a string, or a double to an int.
There are two main types of type casting: implicit and explicit. Implicit type casting is done automatically by the compiler or the interpreter, according to the rules of the programming language. Explicit type casting is done manually by the programmer, using a special syntax or a function.
Here is a little notes that explains type casting in more detail, using examples from different programming languages.
Type Casting in Programming Languages
Type casting, also known as type conversion, is a common technique in programming that allows changing the data type of a value or a variable. It is often necessary to perform type casting when working with different types of data, such as numbers, strings, booleans, etc.
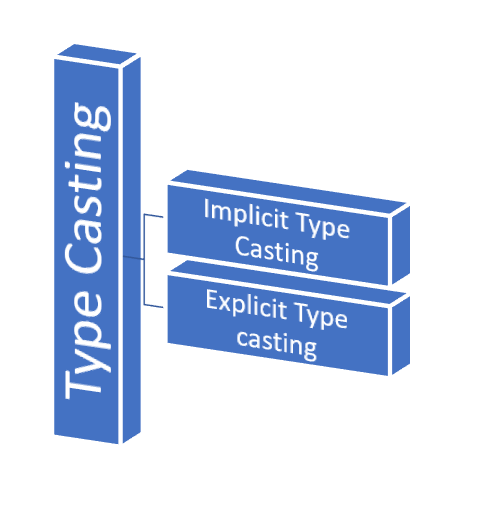
Implicit Type Casting
Implicit type casting is when the compiler or the interpreter automatically converts one data type to another, without any explicit instruction from the programmer. This usually happens when the data type of a value or a variable does not match the expected data type of a function or an expression.
For example, in Python, if we try to add a string and an integer, the interpreter will implicitly convert the integer to a string and concatenate them:
x = "Hello"
y = 5
z = x + y # implicit type casting
print(z) # Hello5
However, implicit type casting does not always work, and sometimes it can lead to errors or unexpected results. For example, in Java, if we try to assign a double value to an int variable, the compiler will throw an error, because it cannot implicitly convert a double to an int:
int x = 10.5; // error: incompatible types
Explicit Type Casting
Explicit type casting is when the programmer explicitly specifies the data type to which a value or a variable should be converted. This can be done using a special syntax or a function, depending on the programming language.
For example, in C, we can use the casting operator (a pair of parentheses with the name of the data type) to convert a value or a variable to another data type:
int x = 10;
double y = 3.14;
int z = (int) y; // explicit type casting
double w = x + y; // implicit type casting
printf("%d\n", z); // 3
printf("%f\n", w); // 13.140000
In the above example, we explicitly cast the double value y to an int value z, using the syntax (int) y. This means that the fractional part of y is discarded, and only the integer part is assigned to z. On the other hand, when we add x and y, the compiler implicitly casts the int value x to a double value, and assigns the result to w.
Another example of explicit type casting is using a built-in function or a method to convert one data type to another. For example, in JavaScript, we can use the Number() function to convert a string to a number, or the toString() method to convert a number to a string:
var x = "10";
var y = 5;
var z = Number(x) + y; // explicit type casting
var w = y.toString() + x; // explicit type casting
console.log(z); // 15
console.log(w); // 510
In the above example, we explicitly cast the string x to a number using the Number() function, and add it to y. We also explicitly cast the number y to a string using the toString() method, and concatenate it with x.
Why Type Casting is Important
Type casting is important because it allows us to manipulate data in different ways, and to avoid errors or bugs in our programs. By using type casting, we can:
- Perform arithmetic operations on different types of numbers, such as integers, floats, doubles, etc.
- Compare values of different data types, such as strings, booleans, etc.
- Pass values of different data types to functions or methods that expect a specific data type.
- Store values of different data types in arrays, lists, dictionaries, etc.
- Convert values of different data types to a common format, such as JSON, XML, etc.
Type casting is a powerful and useful tool in programming, but it should be used with caution and understanding. Sometimes, type casting can result in data loss, precision loss, or unexpected behavior. Therefore, it is important to know the rules and limitations of type casting in each programming language, and to test and debug our code carefully.
Type Casting in Python Programming
Python, being a dynamically typed language, offers great flexibility and ease of use. One of the key features that contribute to its versatility is type casting. Type casting enables developers to convert variables from one data type to another, opening up a plethora of possibilities in terms of data manipulation and program optimization.
In this comprehensive guide, we will delve into the intricacies of type casting in Python, exploring its various aspects and demonstrating how it can be effectively utilized to enhance your coding prowess.
Understanding Type Casting in Python
Type casting, also known as type conversion, refers to the process of converting variables from one data type to another. In Python, this can be achieved through the use of built-in functions or by simply specifying the desired data type during variable assignment.
Implicit Type Casting
Python employs implicit type casting, where the interpreter automatically converts variables from one data type to another as required. This can often lead to unexpected results if not handled carefully. Let’s take a look at an example:
x = 10
y = 5.5
result = x + y
print(result) # Output: 15.5
In this example, Python automatically converts the integer variable x to a floating-point number to perform the addition operation with y.
Explicit Type Casting
While implicit type casting may suffice in many cases, there are scenarios where explicit type casting is necessary to ensure data integrity and avoid errors. Python provides built-in functions for explicit type conversion, such as int(), float(), str(), etc.
x = 10
y = "20"
result = x + int(y)
print(result) # Output: 30
Here, we explicitly convert the string variable y
to an integer using the int() function before performing the addition operation with x
.
Benefits of Type Casting
Type casting offers several advantages in Python programming, including:
- Data Integrity: By converting variables to the appropriate data type, type casting helps maintain data integrity and prevents unexpected behaviors.
- Compatibility: Type casting facilitates interoperability between different data types, allowing for seamless integration within programs.
- Performance Optimization: In certain scenarios, converting variables to more efficient data types can lead to improved performance and resource utilization.
Common Use Cases with Type Casting
Type casting finds widespread applications across various domains in Python programming. Some common use cases include:
User Input Processing
When accepting user input, it’s essential to convert the input data to the desired data type before performing any operations or validations.
age = input("Enter your age: ")
age = int(age) # Convert input to integer
Mathematical Operations
Type casting is often used to ensure uniform data types when performing mathematical operations involving variables of different types.
x = 10
y = "20"
result = x + int(y) # Convert y to integer for addition
File Handling
When reading data from files, type casting allows for proper interpretation and manipulation of the read values.
with open("data.txt", "r") as file:
data = file.read()
number = float(data) # Convert read data to float
Conclusion
In conclusion, mastering type casting in Python is essential for harnessing the full potential of the language. By understanding the principles of type conversion and leveraging it effectively in your code, you can write more robust, efficient, and versatile Python programs.
Whether you’re a beginner or an experienced developer, delving into the nuances of type casting will undoubtedly elevate your Python programming skills to new heights. So embrace the power of type casting and embark on a journey towards becoming a Python virtuoso!
Frequently Asked Questions (FAQ) – Python Type Casting Guide
- Q: What is Python type casting, and why is it important?A: Python type casting, or type conversion, is the process of converting variables from one data type to another. It’s crucial for maintaining data integrity, ensuring compatibility between different data types, and optimizing performance in Python programming.
- Q: How does implicit type casting work in Python?A: Python uses implicit type casting, where the interpreter automatically converts variables from one data type to another as needed. This occurs seamlessly during operations, but it’s important to handle it carefully to avoid unexpected results.
- Q: Can you provide an example of explicit type casting in Python?A: Certainly! Consider the following example where we convert a string variable to an integer using the int() function:
x = 10 y = "20" result = x + int(y)
- Q: What are the benefits of using type casting in Python programming?A: Type casting offers several advantages, including maintaining data integrity, ensuring compatibility between data types, and optimizing performance by converting variables to more efficient types when necessary.
- Q: In what scenarios is explicit type casting necessary?A: Explicit type casting becomes necessary when you want to control the conversion of variables explicitly, ensuring that they are of the desired data type. This is crucial for avoiding errors and maintaining program logic.
- Q: How can type casting be applied to user input processing in Python?A: When processing user input, it’s essential to convert the input data to the desired data type. For example, converting a user’s age input from a string to an integer using the
int()
function. - Q: Are there common use cases for type casting in mathematical operations?A: Yes, type casting is frequently used in mathematical operations to ensure uniform data types when performing calculations involving variables of different types. For instance, converting a string to an integer before addition.
- Q: Does type casting play a role in file handling in Python?A: Absolutely. When reading data from files, type casting allows for the proper interpretation and manipulation of the read values. For instance, converting read data to a float if numerical values are involved.
- Q: Why is mastering type casting important for Python developers of all skill levels?A: Mastering type casting is crucial for writing more robust, efficient, and versatile Python programs. It enhances your ability to manipulate data effectively, making you a more proficient and skilled Python developer.
- Q: How can I start leveraging the power of type casting in Python today?A: Dive into our comprehensive guide and follow the examples provided. Understanding the principles of type conversion and applying them in your code will empower you to harness the full potential of Python type casting.
If you are interested about writing articles then please post your article here without Plagiarism. Link for Guest Post.
Read the Python official documentation here.